Cross-Site Request Forgery (CSRF) is a common security vulnerability that allows attackers to trick authenticated users into executing unwanted actions on a web application. Symfony provides built-in tools to mitigate this risk effectively. In this article, we’ll explore how to secure forms and APIs in Symfony using CSRF protection.
⚠️CSRF attacks exploit the trust a web application has in an authenticated user. An attacker can forge requests on behalf of the user, potentially modifying data, changing user settings, or performing other harmful actions. Symfony prevents CSRF attacks using tokens that validate the authenticity of requests. These tokens ensure that submitted forms and API requests originate from legitimate sources.
📝Enabling CSRF Protection for Forms 📝
Symfony integrates CSRF protection seamlessly with its Form component. To enable it, simply include a CSRF token field in your forms:
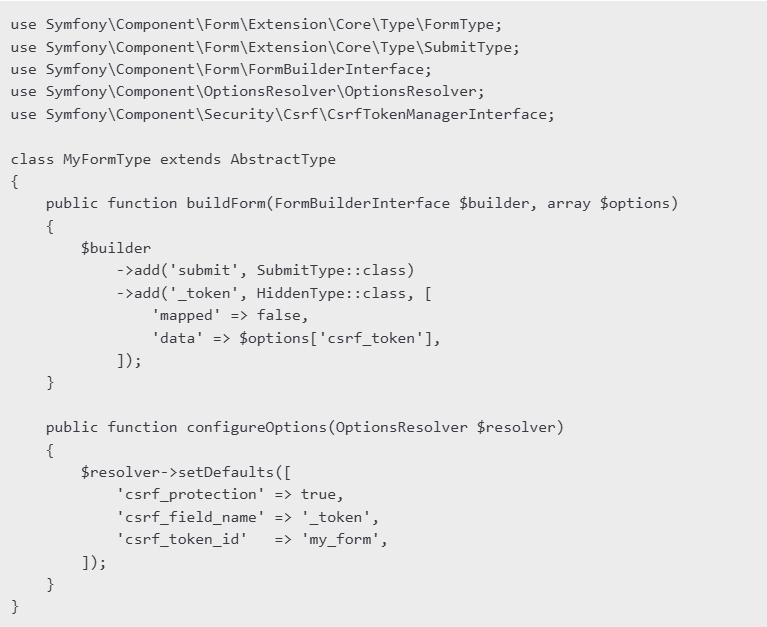
🛡️Validating CSRF Tokens in Controller. When handling form submissions, Symfony automatically checks the CSRF token if csrf_protection
is enabled. However, if manually verifying CSRF tokens, you can use the CsrfTokenManagerInterface
:
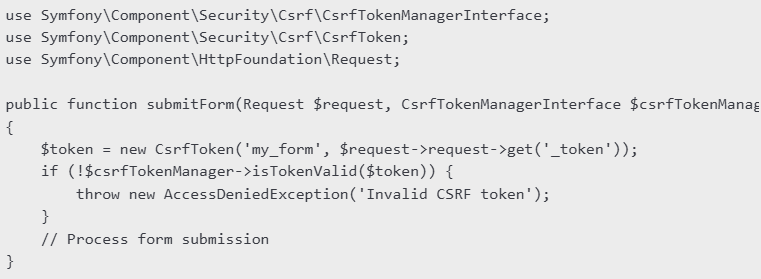
🔐Protecting APIs from CSRF Attacks. APIs are typically stateless and do not maintain sessions like traditional web applications. Therefore, Symfony does not apply CSRF protection to API endpoints by default. However, if your API endpoints modify data and require authentication, you should implement CSRF protection.
🛠️Using CSRF Tokens in API Requests 🛠️
One approach to securing APIs against CSRF attacks is to use CSRF tokens along with user authentication:
- Generate a CSRF token when a user logs in.
- Require the token in each subsequent request.
- Validate the token on the server before processing requests.
Here’s how you can generate and validate CSRF tokens in an API:
🔑 Generate a CSRF Token
public function getCsrfToken(CsrfTokenManagerInterface $csrfTokenManager): JsonResponse
{
$token = $csrfTokenManager->getToken('api_request');
return new JsonResponse(['csrf_token' => $token->getValue()]);
}
🔑Validate CSRF Token in API Requests
public function handleApiRequest(Request $request, CsrfTokenManagerInterface $csrfTokenManager)
{
$token = new CsrfToken('api_request', $request->headers->get('X-CSRF-Token'));
if (!$csrfTokenManager->isTokenValid($token)) {
return new JsonResponse(['error' => 'Invalid CSRF token'], 403);
}
// Process API request
}
Besides CSRF tokens, you can enhance security by:
- Using SameSite Cookies: Configure cookies with
SameSite=strict
to prevent CSRF attacks from third-party websites. - Requiring Authentication Headers: Enforce authentication mechanisms like JWT or OAuth to verify API requests.
- Validating Origin Headers: Ensure API requests originate from trusted sources by checking the
Origin
andReferer
headers.
CSRF protection is crucial for securing Symfony applications, particularly when dealing with forms and authenticated API requests. By leveraging Symfony’s built-in CSRF token management, you can ensure that only legitimate requests are processed, safeguarding your users from potential attacks. Implement these security best practices to fortify your application against CSRF threats!
Contact us now🚀