In web development, performance is key. As applications grow, so does the size of their databases, which can lead to performance issues. One common use case is when you need to keep a limited number of records in the database—such as keeping the latest 100 blog posts or the most recent entries in a log table. But what happens when these records exceed the limit? This is where Laravel’s elegant solutions come into play.
Why Limit Records?
Before we dive into the how, let’s take a quick look at why you’d want to keep your database records limited:
- Performance: The more records your database holds, the slower your queries will become over time, especially when retrieving the most recent data.
- Storage: Unnecessary records can accumulate, taking up valuable storage space.
- Cost: In cloud environments, where you’re billed based on data storage, it’s important to keep the database lean.
By keeping your records limited, you can ensure that your database operates efficiently and stays manageable.
Laravel’s Approach to Managing Record Limits
Laravel offers several elegant ways to achieve this goal. Below are some of the most common strategies you can use to ensure your database doesn’t become bloated over time.
✒️ Using Model::latest()->take()
to Limit Results
If your goal is simply to retrieve a fixed number of records (for example, the latest 100 blog posts), you can use the latest()
method in combination with take()
to limit the number of records:

This query will grab the latest 100 posts from your posts
table. While this is great for limiting the results you retrieve, it doesn’t address removing old records to keep the table size manageable.
✒️ Pruning Old Records Automatically with Eloquent
and a Scheduler
One of the most efficient ways to handle a fixed number of records is to delete older records automatically. For example, let’s say you only want to keep the latest 100 blog posts in your posts
table. You can use Laravel’s task scheduling to periodically delete records exceeding the limit.
Here’s how you can set up automatic pruning:
- Create an Artisan Command: First, create an Artisan command to handle the pruning logic. Run:

✒️ Define the Command Logic: In the handle()
method of the generated command, use the following code:
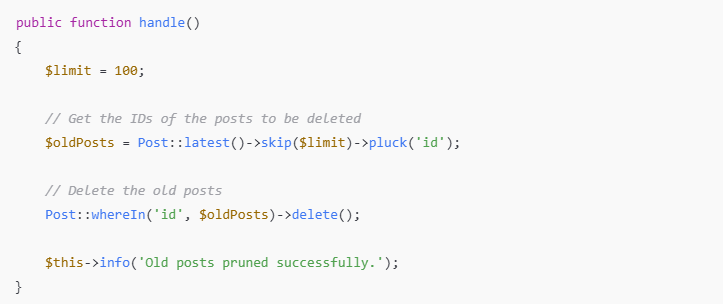
This code ensures that once the number of posts exceeds 100, the older posts are deleted, keeping the database lean and performant.
✒️ Schedule the Command: Add the command to Laravel’s scheduler by editing the app/Console/Kernel.php
file. In the schedule()
method, add:

This will run the pruning task daily. You can adjust the frequency based on your needs (e.g., hourly, weekly).
✒️ Run the Scheduler: To make the scheduler run automatically, set up a cron job on your server:

✒️ Using a Database Trigger (Advanced)
For more advanced users, database triggers can be a great option. A trigger can be set to automatically delete old records when a new record is inserted. However, this method depends on the database you’re using and can be a bit trickier to implement in Laravel. Here’s an example for MySQL:
- Create the Trigger: In your migration or directly in MySQL, you can set up a trigger that deletes the oldest records when a new record is added:
DELIMITER $$
CREATE TRIGGER limit_posts BEFORE INSERT ON posts
FOR EACH ROW
BEGIN
DECLARE record_count INT;
-- Count the current number of records
SELECT COUNT(*) INTO record_count FROM posts;
-- If more than 100 posts exist, delete the oldest one
IF record_count > 100 THEN
DELETE FROM posts WHERE id NOT IN (SELECT id FROM posts ORDER BY created_at DESC LIMIT 100);
END IF;
END$$
DELIMITER ;
- With this approach, every time a new post is added, the database automatically checks if there are more than 100 posts. If so, it deletes the oldest posts. This is highly efficient but also more database-dependent.
✒️ Using Soft Deletes for More Control
Sometimes, instead of fully deleting records, you might want to “soft delete” them, meaning they are marked as deleted without actually removing them from the database. This allows you to keep the record for future reference but not include it in your queries.
Laravel’s SoftDeletes
feature can help you achieve this:
- Enable Soft Deletes: In your
Post
model, add theSoftDeletes
trait:
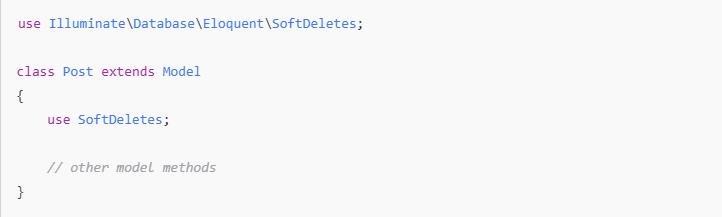
- Prune Soft Deleted Records: You can then use the
forceDelete()
method to permanently remove records that have been soft deleted. For example, you could create a command to prune records marked as soft deleted:
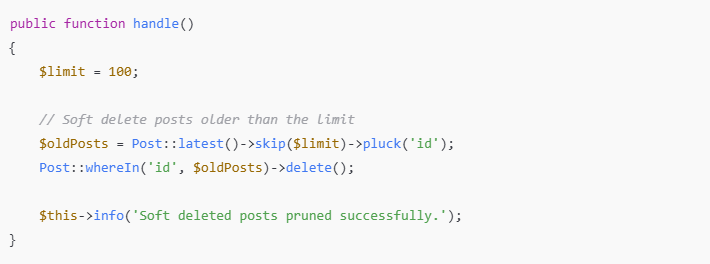
✒️Implementing Limits Using Laravel Collections
If you’re retrieving the data and then manipulating it within Laravel, consider limiting the records directly using Laravel’s collection methods:

This approach is suitable for scenarios where the logic is applied to the retrieved data but doesn’t affect the database directly.
In Laravel, there are several ways to limit the number of records in your database while ensuring optimal performance. Whether you’re using built-in Eloquent methods like take()
or setting up automatic pruning with Artisan commands, Laravel offers the flexibility to easily implement these techniques. By keeping your records in check, you can improve your application’s speed, reduce storage costs, and ensure your database remains responsive.
Choose the method that best fits your needs, and let Laravel handle the heavy lifting for you!