Creating a simple URL shortener can be an interesting project to sharpen your PHP and MySQL skills. With this tutorial, you will learn to build a basic URL shortening tool using PHP and MySQLi in just four steps.
☞ Step 1: Set Up Your Project Environment
First, set up the basic project structure. Create a folder named url-shortener
in your local server’s document root. Inside this folder, create the following files:
index.php
— for the main application logic and URL shortening form.redirect.php
— to handle redirection when a short URL is accessed.db.php
Make sure you have a working local server with PHP and MySQL support (e.g., XAMPP, MAMP, or LAMP).
Database Creation
Start by creating a database and a table in MySQL:
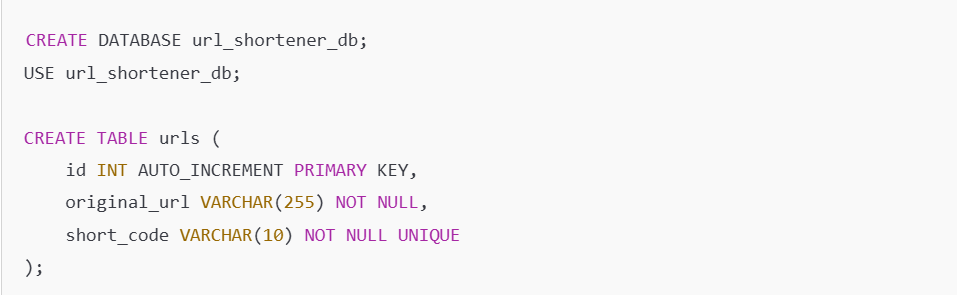
☞ Step 2: Establish Database Connection (db.php
)
In db.php
, write the code for establishing a connection to your MySQL database using MySQLi
.
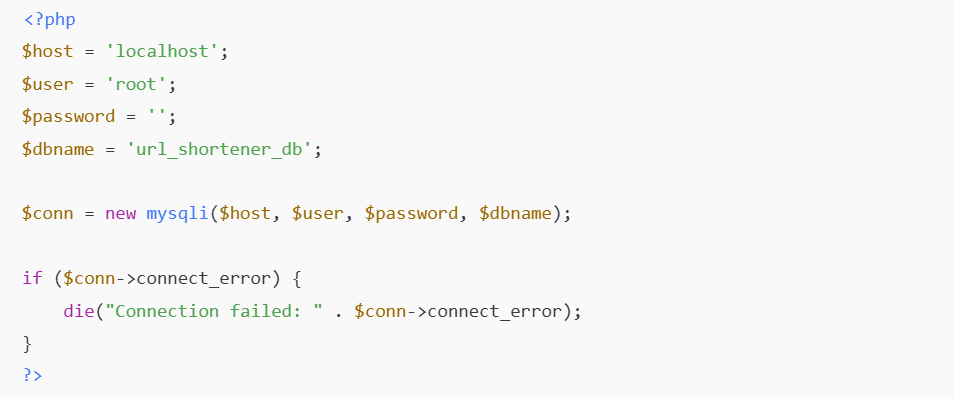
Ensure the database credentials ($user
, $password
, $dbname
) match your setup.
☞ Step 3: Build the URL Shortening Logic (index.php
)
In index.php
, create a form to accept the long URL and handle the URL shortening process.
<?php
include 'db.php';
function generateShortCode($length = 6) {
return substr(str_shuffle("abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"), 0, $length);
}
if (isset($_POST['submit'])) {
$original_url = $conn->real_escape_string($_POST['url']);
$short_code = generateShortCode();
// Check if URL already exists
$result = $conn->query("SELECT * FROM urls WHERE original_url = '$original_url'");
if ($result->num_rows > 0) {
$row = $result->fetch_assoc();
$short_code = $row['short_code'];
} else {
$conn->query("INSERT INTO urls (original_url, short_code) VALUES ('$original_url', '$short_code')");
}
$short_url = "http://localhost/url-shortener/redirect.php?c=$short_code";
echo "Shortened URL: <a href='$short_url'>$short_url</a>";
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>URL Shortener</title>
</head>
<body>
<h2>Enter a URL to shorten:</h2>
<form method="post" action="">
<input type="url" name="url" required placeholder="Enter a long URL" style="width: 300px;">
<button type="submit" name="submit">Shorten</button>
</form>
</body>
</html>
This script:
- Takes a URL input and generates a unique shortcode.
- Checks if the URL already exists in the database to avoid duplication.
- Inserts new records if the URL is not found.
☞ Step 4: Handle URL Redirection (redirect.php
)
redirect.php
will be used to process the short URL and redirect users to the original URL.
<?php
include 'db.php';
if (isset($_GET['c'])) {
$code = $conn->real_escape_string($_GET['c']);
$result = $conn->query("SELECT * FROM urls WHERE short_code = '$code'");
if ($result->num_rows > 0) {
$row = $result->fetch_assoc();
header("Location: " . $row['original_url']);
exit();
} else {
echo "Short URL not found!";
}
} else {
echo "No short code provided!";
}
?>
This script:
- Checks for the
c
query parameter. - Searches the database for the corresponding original URL.
- Redirects to the original URL if found; otherwise, displays an error message.
With these four steps, you’ve built a simple yet functional URL shortener using PHP and MySQLi. This project covers essential concepts such as form handling, database operations, and URL redirection, giving you a practical application for developing further projects.
Feel free to expand on this foundation by adding features like custom shortcodes, tracking click counts, or creating a user-friendly interface.
If you have any questions about your project, please write to us 🚀🚀🚀
We are happy to help🤝