Becoming a senior JavaScript React developer involves more than just mastering the basics. It requires a deep understanding of the React ecosystem and best practices for building scalable, maintainable, and efficient applications.
Let’s take a deep dive into these advanced techniques, illustrated with code examples, and supercharge your React skills.
➣Optimize Rendering:
🠒Use React’s PureComponent or memo() function to optimize rendering by preventing unnecessary re-renders of components.
🠒Implement shouldComponentUpdate lifecycle method to fine-tune rendering conditions and prevent unnecessary updates.
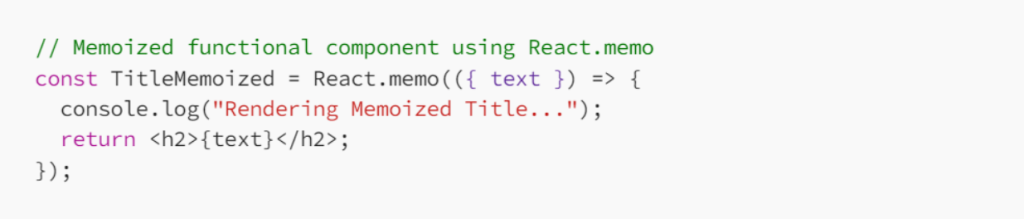
➣Virtualize Long Lists: For long lists or large datasets, use virtualization libraries like React Virtualized or React Window to render only the items visible on the screen, improving performance and reducing memory usage.
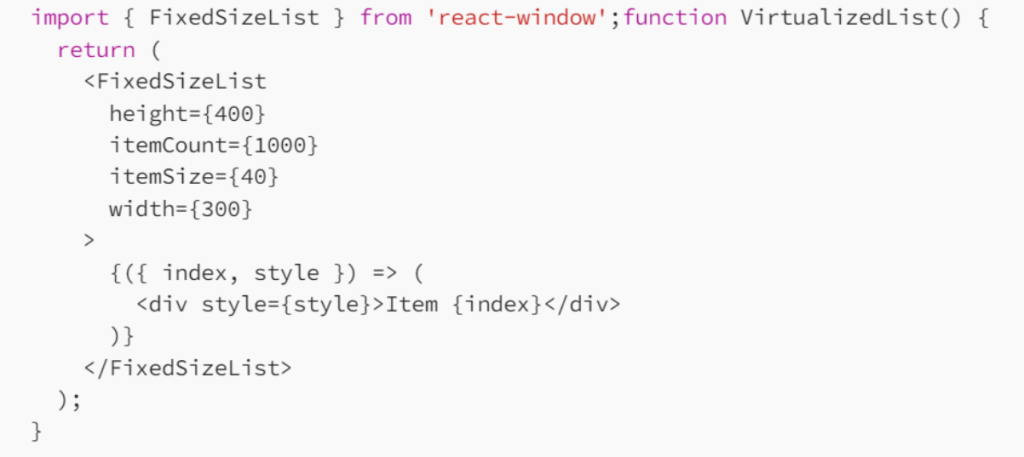
➣Code Splitting: Employ code splitting techniques to split your application into smaller chunks that are loaded on demand, reducing the initial load time and improving overall performance. React.lazy and dynamic imports are helpful for this purpose.
const MyLazyComponent = React.lazy(() => import('./MyLazyComponent'));function App() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<MyLazyComponent />
</Suspense>
</div>
);
}
➣Memoization: Utilize memoization techniques, such as caching expensive function results using libraries like memoize-one or useMemo hook, to optimize performance by avoiding unnecessary computations.
➣Bundle Optimization: Optimize your webpack or bundler configuration to reduce bundle size by eliminating dead code, using tree shaking, and minimizing dependencies. Tools like Webpack Bundle Analyzer can help identify optimization opportunities.
# Install the analyzer package
npm install --save-dev webpack-bundle-analyzer# Update npm scripts
"scripts": {
"analyze": "webpack --profile --json > stats.json && webpack-bundle-analyzer stats.json"
}
➣Debounce and Throttle: Use debounce and throttle techniques to control the frequency of function invocations in response to events like window resize or input changes, preventing excessive renders and improving performance.
import React, { useState } from 'react';
const App = () => {
const [debouncedValue, setDebouncedValue] = useState('');
const [throttledValue, setThrottledValue] = useState('');
// Debounce function
const debounce = (func, delay) => {
let timer;
return function (...args) {
clearTimeout(timer);
timer = setTimeout(() => func.apply(this, args), delay);
};
};
// Throttle function
const throttle = (func, limit) => {
let lastFunc;
let lastRan;
return function (...args) {
const context = this;
if (!lastRan) {
func.apply(context, args);
lastRan = Date.now();
} else {
clearTimeout(lastFunc);
lastFunc = setTimeout(function () {
if (Date.now() - lastRan >= limit) {
func.apply(context, args);
lastRan = Date.now();
}
}, limit - (Date.now() - lastRan));
}
};
};
// Debounced input change handler
const handleDebouncedChange = debounce((value) => {
setDebouncedValue(value);
}, 500);
// Throttled input change handler
const handleThrottledChange = throttle((value) => {
setThrottledValue(value);
}, 500);
const handleChange = (e) => {
const { value } = e.target;
// Use the debounce or throttle handler here
handleDebouncedChange(value);
handleThrottledChange(value);
};
return (
<div>
<input type="text" onChange={handleChange} placeholder="Type something..." />
<div>
<h2>Debounced Value:</h2>
<p>{debouncedValue}</p>
</div>
<div>
<h2>Throttled Value:</h2>
<p>{throttledValue}</p>
</div>
</div>
);
};
export default App;
In this example:
debounce
function creates a debounced version of a function that delays its execution until after a certain amount of time has passed since the last time it was invoked.throttle
function creates a throttled version of a function that limits the rate at which a function can be invoked.- We use these functions to debounce and throttle the
handleChange
function, which is triggered on every input change event. handleDebouncedChange
andhandleThrottledChange
are the debounced and throttled versions of thehandleChange
function, respectively.- The state variables
debouncedValue
andthrottledValue
hold the latest values after debounce and throttle.
➣Server-Side Rendering (SSR): Implement server-side rendering (SSR) for React applications to improve initial load times, enable better SEO, and provide a more consistent user experience, especially for users on slow networks or devices.
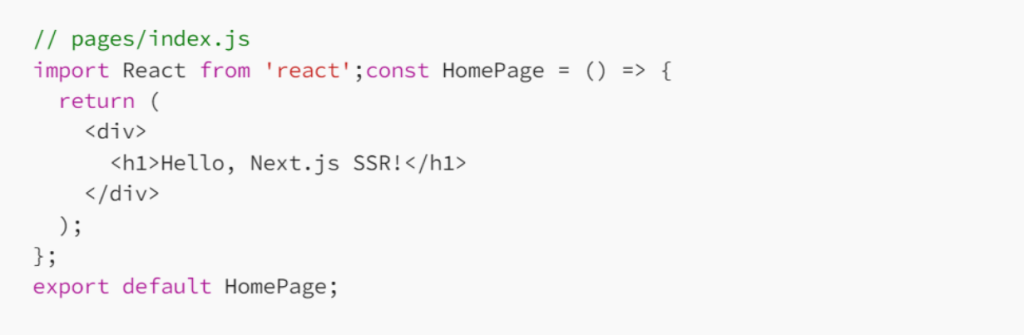
By incorporating these performance tips into your development workflow, you can optimize your React applications for better scalability, responsiveness, and overall user experience, showcasing your expertise as a senior JavaScript React developer.
Fill-in the form below to reach out to us with your project👇