Code smells are indicators of potential issues in a codebase that can lead to maintenance challenges, bugs, and overall decreased code quality. In PHP development, recognizing and eliminating code smells is crucial for creating maintainable, efficient, and robust applications. This practical guide will explore common PHP code smells and provide actionable strategies to refactor and improve your code.
1. Duplicated Code
Code Smell: Repetitive blocks of code scattered throughout the application.
Solution: Encapsulate the duplicated code into functions or classes. This not only reduces redundancy but also makes the code easier to maintain. Utilize inheritance or composition to share common functionality.
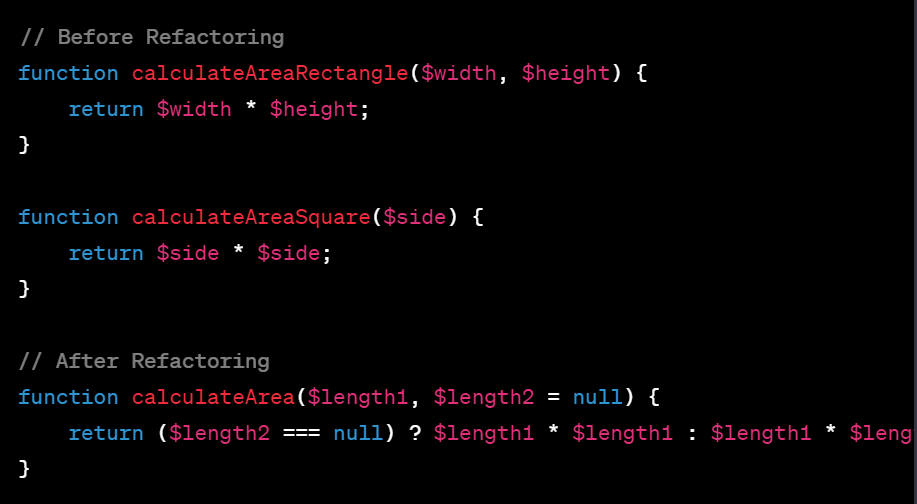
2. Long Methods
Code Smell: Functions or methods that are excessively long and perform multiple tasks.
Solution: Break down long methods into smaller, focused functions. This enhances code readability, promotes reusability, and simplifies testing.
// Before Refactoring
function processOrder($order) {
// ... 100 lines of code ...
}
// After Refactoring
function validateOrder($order) {
// ... validation logic ...
}
function calculateTotal($order) {
// ... calculation logic ...
}
function updateInventory($order) {
// ... inventory update logic ...
}
function processOrder($order) {
validateOrder($order);
calculateTotal($order);
updateInventory($order);
}
3. God Class
Code Smell: A class that does too many things and has too many responsibilities.
Solution: Apply the Single Responsibility Principle (SRP) by breaking down the class into smaller, specialized classes. Each class should have a single responsibility.
// Before Refactoring
class OrderProcessor {
public function processOrder($order) {
// ... 200 lines of code ...
}
}
// After Refactoring
class OrderValidator {
public function validateOrder($order) {
// ... validation logic ...
}
}
class OrderCalculator {
public function calculateTotal($order) {
// ... calculation logic ...
}
}
class InventoryUpdater {
public function updateInventory($order) {
// ... inventory update logic ...
}
class OrderProcessor {
public function processOrder($order) {
$validator = new OrderValidator();
$calculator = new OrderCalculator();
$updater = new InventoryUpdater();
$validator->validateOrder($order);
$calculator->calculateTotal($order);
$updater->updateInventory($order);
}
}
4. Magic Numbers
Code Smell: Direct usage of numeric literals without clear explanation.
Solution: Replace magic numbers with named constants or variables to improve code readability and maintainability.
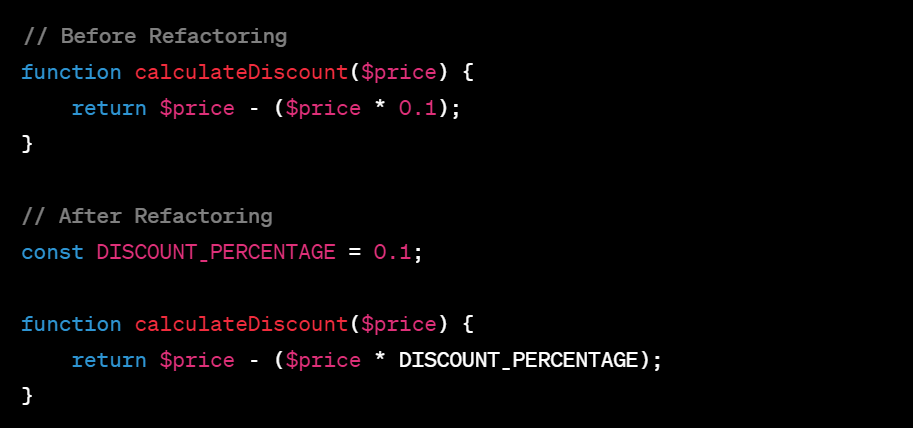
5. Inconsistent Naming
Code Smell: Variables, functions, or classes with inconsistent or unclear naming conventions.
Solution: Adopt a consistent naming convention and ensure that names are descriptive and convey the purpose of the entity.
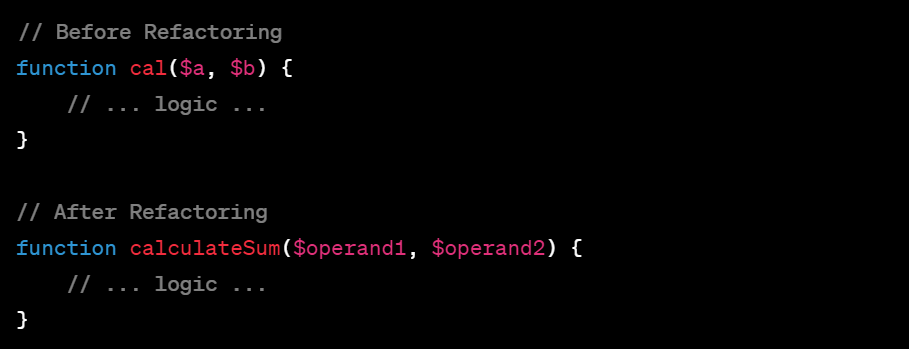
6. Inconsistent Naming
Code Smell: Variables, functions, or classes with inconsistent or unclear naming conventions.
Solution: Adopt a consistent naming convention and ensure that names are descriptive and convey the purpose of the entity.
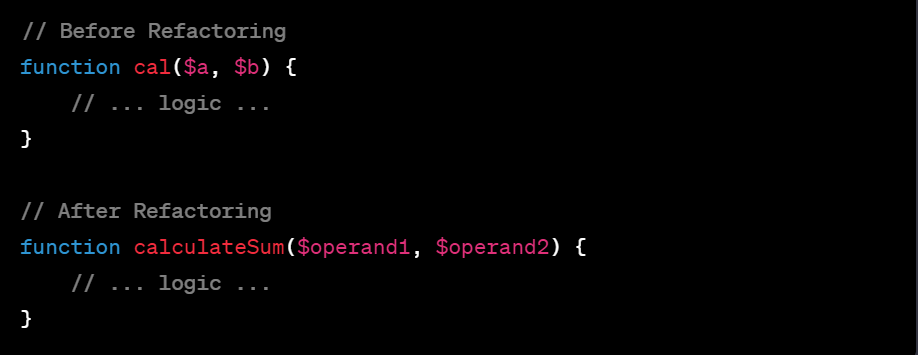
Recognizing and eliminating code smells is an essential aspect of maintaining a healthy and scalable PHP codebase. By applying the principles of clean code and refactoring, developers can enhance code quality, readability, and maintainability. Regularly reviewing and refactoring code for common smells leads to a more robust and efficient application development process.
Fill-in the form below to reach out to us with your project👇