React is a game-changer for building interactive UIs, but even the most elegant libraries have quirks. One recurring challenge is JSX’s conditional rendering—essential for controlling what appears on the screen. While React provides tools like if
statements, ternary operators, and &&
, they can quickly lead to messy, unreadable, and difficult-to-maintain code. Let’s dive into why this happens and how we can rethink conditional rendering in React.
The Challenges of JSX Conditional Rendering💡
1. Readability vs. Complexity
A simple condition is fine:
{isLoggedIn ? <Welcome /> : <Login />}
But as logic grows more complex, ternaries and &&
operators can make JSX unreadable:
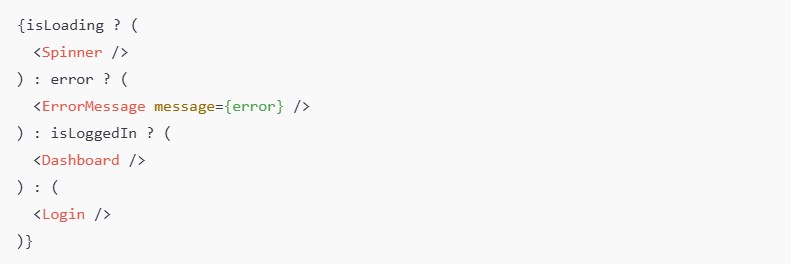
2. Duplication of Logic
Often, developers end up duplicating conditions or mixing concerns. For example, checking the same condition across multiple components increases maintenance overhead:
{isAdmin && <AdminPanel />}
{!isAdmin && <UserDashboard />}
Any updates to isAdmin
logic must be synchronized across all related components.
3. Hard-to-Debug Errors
Nest conditions poorly, and you risk introducing subtle bugs. For example, overusing the &&
operator can unintentionally render falsy values: {isAvailable && <div>{data.name}</div>}
If data.name
is null
or 0
, React will render these unintended values.
Revolutionizing Conditional Rendering🚀
To tackle these pain points, here are some approaches to revolutionize conditional rendering in React.
1. Custom Components for Conditional Rendering
Encapsulating logic into reusable components improves readability and modularity.
Example: RenderIf
Component
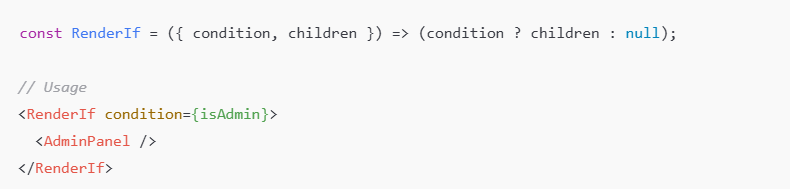
This abstracts the conditional logic, making your JSX easier to read and maintain.
2. Declarative Conditional Mapping
Use JavaScript objects to map conditions to components. This eliminates nested logic chains.
Example: Condition Map
const renderContent = {
isLoading: <Spinner />,
error: <ErrorMessage message={error} />,
isLoggedIn: <Dashboard />,
};
const contentKey = isLoading ? 'isLoading' : error ? 'error' : isLoggedIn ? 'isLoggedIn' : null;
return contentKey ? renderContent[contentKey] : <Login />;
By centralizing conditions, the logic is easier to debug and extend.
3. Hooks for Complex Conditions
Custom hooks can encapsulate logic while providing flexibility.
Example: useConditionalRender
Hook
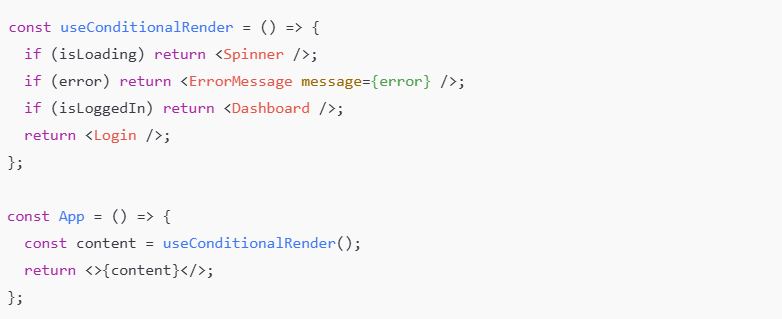
This isolates conditional logic from your JSX, improving both clarity and maintainability.
4. Library-Based Solutions
Consider libraries like clsx or classnames for conditional class management, or create a utility for rendering logic.
Example: Utility Function
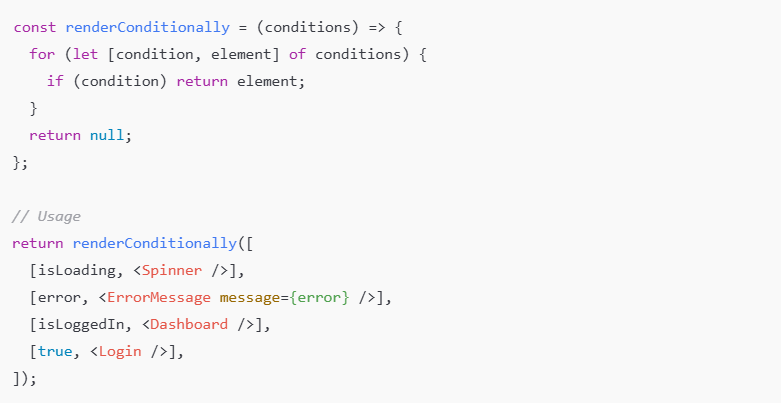
This pattern minimizes nested logic and ensures a clean rendering flow.
JSX’s native conditional rendering tools work, but they often result in bloated, tangled code. By leveraging custom components, declarative patterns, hooks, or utility functions, we can streamline conditional rendering and make React codebases more readable, maintainable, and bug-free.
Conditional rendering doesn’t have to be a conundrum—it can be an opportunity to create beautifully expressive and clean UIs. Revolutionize your approach today!
Happy coding🚀🚀🚀